Python | Page 3
Explore our comprehensive tutorials on Python. Master skills and stay updated with the latest tech insights.
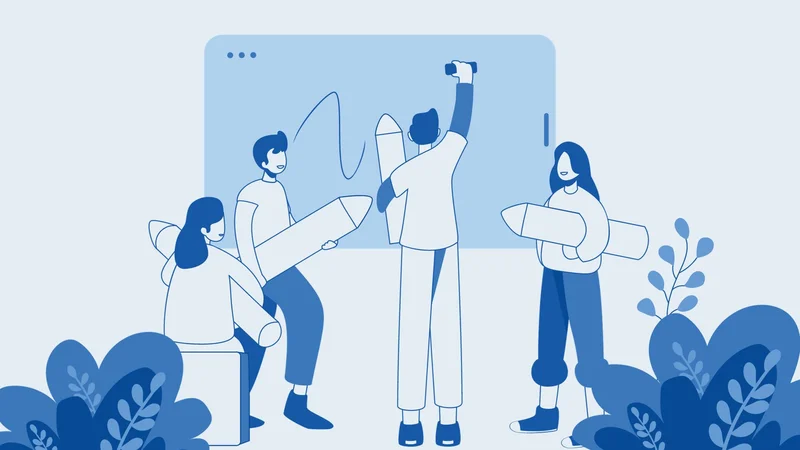
Making HTTP Requests in python
Python In the realm of Python programming, the ability to make HTTP requests is crucial for interacting with web servers and consuming web services. While the popular `requests` library offers a convenient way to accomplish this task, there may be scenarios where you prefer to avoid external... Read more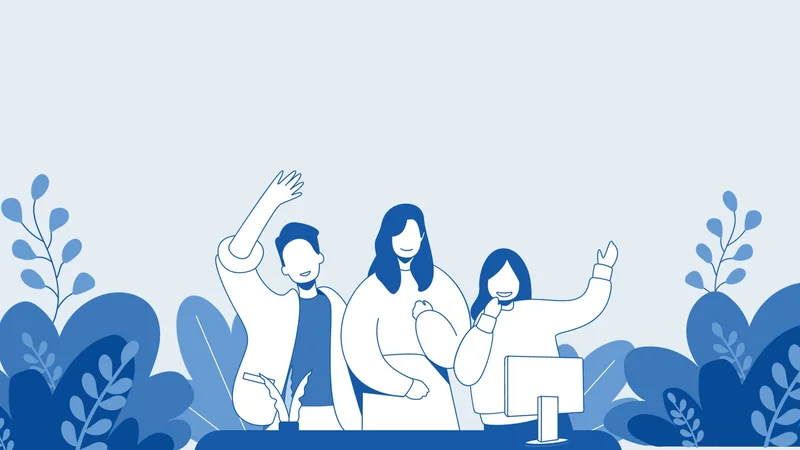
Concurrent Programming with Asyncio
Python Python's asyncio library provides a powerful toolset for achieving concurrency in your applications. Whether you're building web servers, network clients, or any other I/O-bound tasks, asyncio can significantly boost performance by executing multiple operations concurrently. In this article,... Read more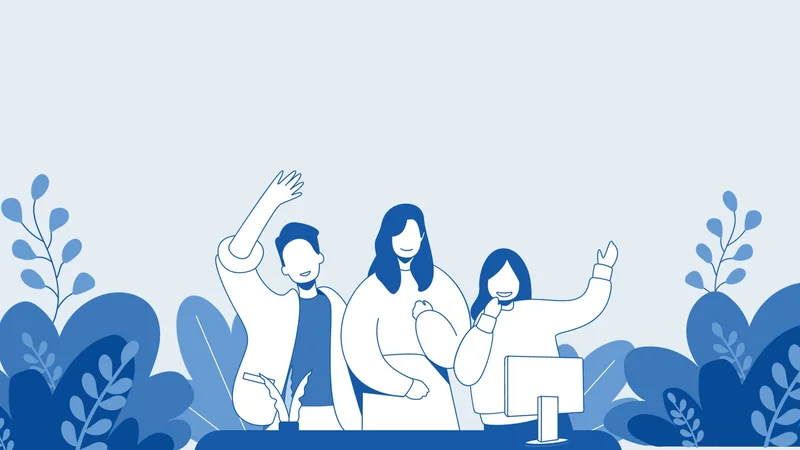
How to achieve Concurrency and Parallelism in Python
Python Have you ever worked on concurrent programming in Python? If not don't worry. In this tutorial, we are going to differentiate between concurrency and Parallelism in Python, how to achieve them, and get the ability to choose the best approach whenever needed. As Python developers, we have to... Read more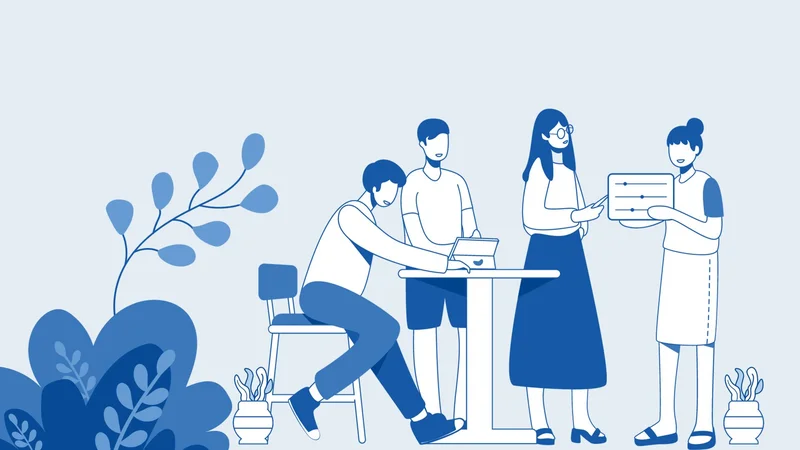
Threading VS Multiprocessing in Python
Python In Python, when it comes to executing tasks concurrently, threading and multiprocessing are two popular options. Each has its strengths and weaknesses, making them suitable for different scenarios. Understanding the differences between threading and multiprocessing is crucial for optimizing... Read more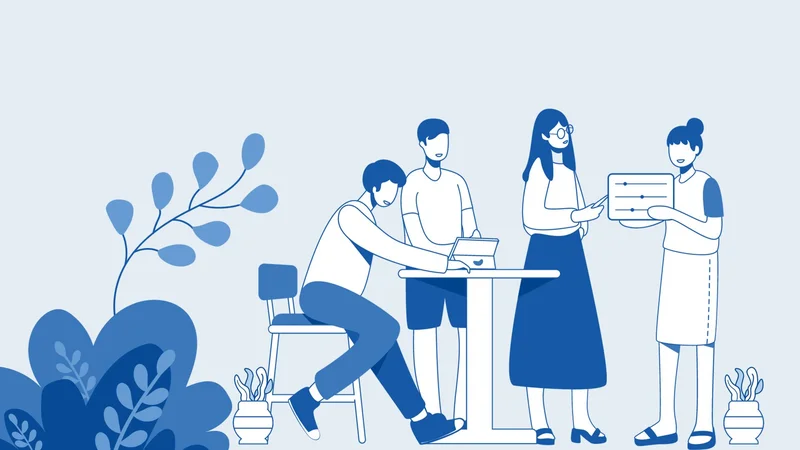
Python Regular Expressions Pattern Matching
Python Regular expressions, often abbreviated as regex or regexp, are powerful tools for pattern matching and text manipulation in Python. They provide a concise and flexible means of searching, extracting, and replacing specific patterns within strings. In this article, we'll delve into the... Read more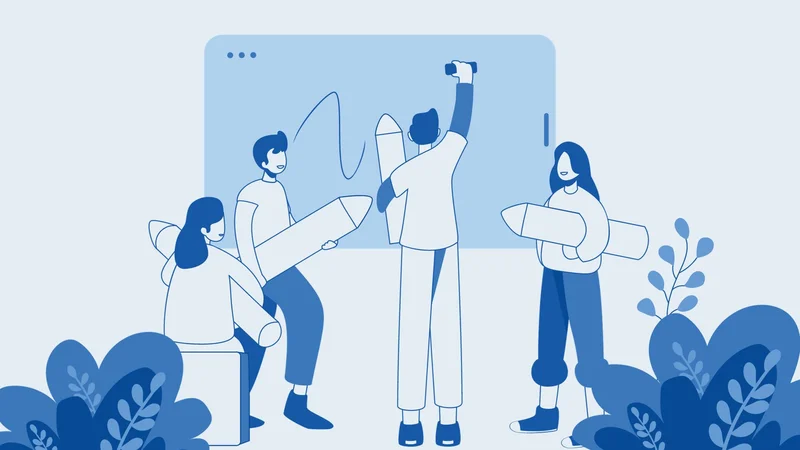
Python Regular Expressions in Text Processing
Python Python regular expressions (regex) serve as indispensable tools for extracting, searching, and manipulating text data efficiently. For those who delve into the realm of programming, understanding regex unlocks a plethora of possibilities in handling textual information. Python, with its built-in... Read more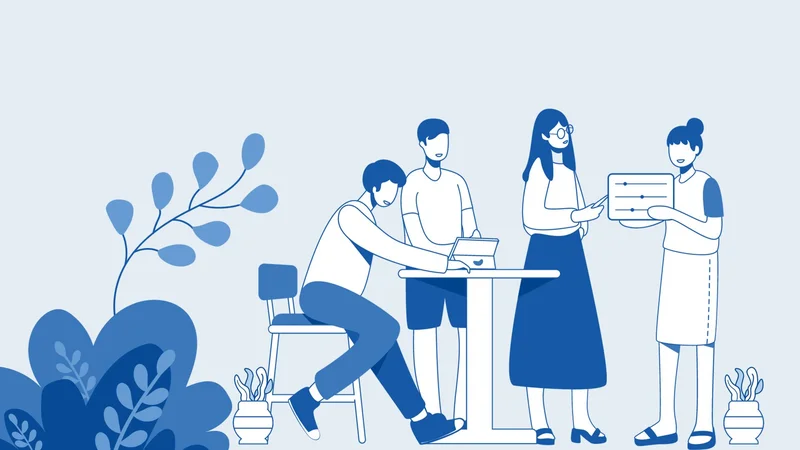
Python Debugging Techniques and Tools
Python Python programming offers immense flexibility and power, but debugging errors can be a daunting task for beginners and seasoned developers alike. Fortunately, with the right techniques and tools at your disposal, you can efficiently identify and resolve bugs in your Python code. In this article,... Read more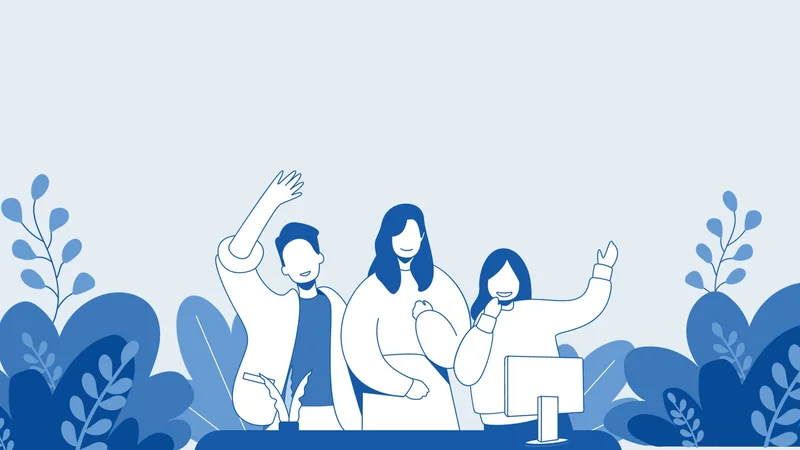
Python Exception Handling
Python In Python programming, exceptions play a crucial role in handling errors that may occur during the execution of a program. Understanding how to effectively handle exceptions can greatly improve the robustness and reliability of your Python code. This article will explore the fundamentals of... Read more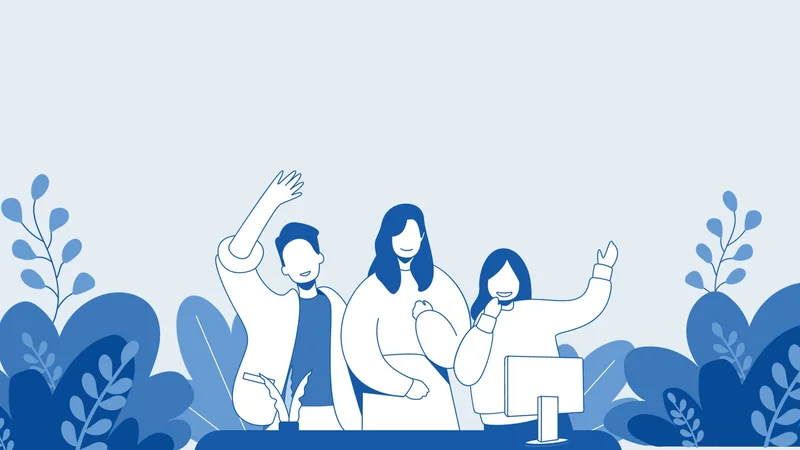