Git commands explained with examples
Understanding Git commands and their practical applications is essential for effective version control in project management. Git serves as a valuable tool to oversee alterations within your project, enhancing team productivity through streamlined change tracking. The command-line interface provided by Git offers a user-friendly means to efficiently manage these changes.
- What is git CLI?
- Git working directory vs staging area vs local repository
- What is git origin?
- Git log commands
- Git branch command
- How to change the global git user?
- Git push command
- Git pull command
- Git fetch command
- Git merge command
- Git checkout command
- Git reset command
- Git revert command
- Git diff command
- Tools available for git
- How to pull a git repo with large files?
- TIPS
- Conclusion
- FAQ
What is git CLI?
Git CLI is a tool where you can run git commands to manage your code changes. There are so many git commands available. But in this article, we are going to focus on the most important git commands that developers use frequently for day-to-day development activities.
Git working directory vs staging area vs local repository
Git creates three areas for each project in the local machine. We call them working area, staging or indexing area, and local repository. The main advantage of separating out a git project into those areas is increasing code manageability.
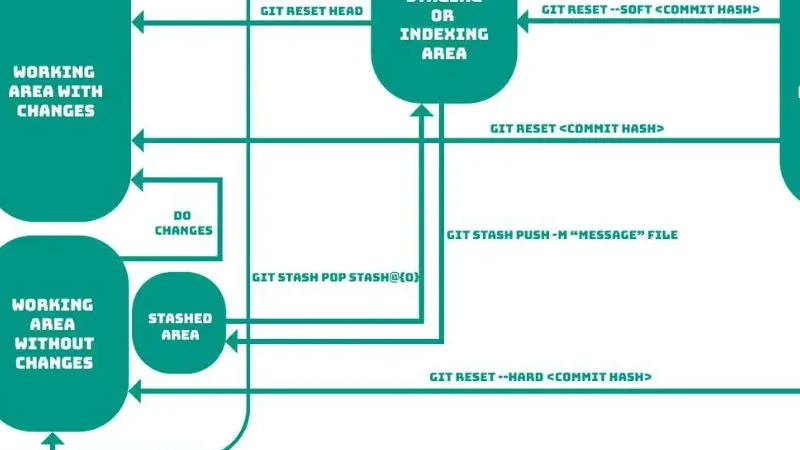
What is git origin?
Origin is the short name that represents the remote server. In another way, the origin is the short form of the URL of the remote server.
List all remote server URLs with their short names
Command
git remote -v
Example
git remote -v
Result
origin https://<username>:<toke>@github.com/SmadusankaB/react-refresh-page.git (fetch)
origin https://<username>:<toke>@github.com/SmadusankaB/react-refresh-page.git (push)
Add a new remote URL with the short name into a git project
Command
git add <url_short_name> <remote_url>
Example
git remote add origin https://github.com/SmadusankaB/Material-Side-Navbar-In-Angular.git
Git log commands
Head shows where exactly you are in your commit history of a given branch.
Command
git log
Example
git log
Result
commit 9cc06fefc3983846e1e9665b881bb4ef68e33c02 (HEAD -> main, origin/main)
Author: SeNewGit <[email protected]>
Date: Sun Jan 8 00:09:25 2023 +0530
first commit
Git branch command
Branches isolate your code changes. The git branch command lists available branches in a given repository.
List out available branches in a given repository
Command
git branch
Example
git branch
Result
* main
reate a new branch from the current branch in a given repository
Command
git checkout -b <branch_name>
Example
git checkout -b new-branch
Result
Switched to a new branch 'new-branch'
Checkout to existing branch in a given repository
Command
git checkout <branch-name>
Example
git checkout main
Result
Switched to branch 'main'
Your branch is up to date with 'origin/main'.
Soft delete a given branch from a repository
-d stands for soft-delete. Soft-delete means, if there are unmerged changes in the branch, git doesn’t delete that branch.
Command
git branch -d <branch_name>
Example
git branch -d new-branch
Result
Deleted branch new-branch
Hard delete a given branch from a repository
-D stands for hard delete. Hard-delete means, if there are unmerged changes in the branch, git doesn’t care and deletes the branch including those changes.
Command
git branch -D <branch_name>
Example
git branch -D new-branch
Result
Deleted branch new-branch (was 9cc06fe)
How to change the global git user?
You can run the git config --global –edit
command in the terminal to change the global git user. After running the command, you will be able to see the [user] block and [credentials] block in the git config file. Update the username, and password and save the changes.
-
Open the global git configurations file:
git config --global --edit
-
Change the username, and password in the global git config file and save it.
Git push command
How to push a previous commit in Git?
There are 3 steps that you need to be performed to be able to push a previous commit. Get the hashed values by running “git log —oneline”. It gives the short format of the hashed value. Copy the corresponding commits hash-value and then run git --hard reset <commit_hash>
. Finally, push that commit into the remote branch with the —force option.
-
List commits hashed in history:
git log --oneline
-
Reset commit:
git reset --hard <commit_hash>
-
Push the selected commit into the remote repository:
git push —force origin <branch_name>
Push a branch in git
The git push command is used to push all changes or specified branches in the local repository to the remote repository. The command syntax is shown below.
Command
git push <url_short_name> <branch_name>
Example
git push origin main
Result
Enumerating objects: 7, done.
Counting objects: 100% (7/7), done.
Delta compression using up to 8 threads
Compressing objects: 100% (4/4), done.
Writing objects: 100% (4/4), 362 bytes | 362.00 KiB/s, done.
Total 4 (delta 3), reused 0 (delta 0), pack-reused 0
remote: Resolving deltas: 100% (3/3), completed with 3 local objects.
To https://github.com/SmadusankaB/react-refresh-page.git
9cc06fe..28f7985 main -> main
Git pull command
How to pull a previous commit in Git?
Sometimes you might need to pull a previous commit from the remote repository. In that case, you can run the reset command with the —hard option. Git log —oneline command lists all commit hashes in short format. Select the commit hash that you want to pull and run the git reset command along with the commit hash.
-
List commits hashed in history:
git log –oneline
-
Pull a commit:
git reset --hard <commit_hash>
Pull branches in git
The git pull command does 2 things. First, it gets all changes or a specified branch from the remote repository. And then it will merge those changes with the local working area. The command syntax is shown below.
Command
git pull <url_short_name> <branch_name>
Example
git pull origin main
Result
From https://github.com/SmadusankaB/react-refresh-page
* branch main -> FETCH_HEAD
Already up to date.
Git fetch command
git fetch command is almost similar to the git pull command. But it doesn’t merge changes with the local working area. The command syntax is given below.
Note: git pull is equal to git fetch + git merge.
Command
git fetch <url_short_name> <branch_name>
Example
git fetch origin main
Result
* branch main -> FETCH_HEAD
How to get a new branch from the remote repository
In a situation where you have to get a new branch from the remote repository to the local, a new branch should be created with the same name as in the remote. Git checkout -b command will create a new branch in the local repository.
-
Create a new branch locally:
git checkout -b <branch_name_in_the_remote_repositoty>
-
Pull the new branch from the remote repository:
git pull origin <branch_name_in_the_remote_repositoty>
Note: Aother way of getting a new branch from the remote repository is, first fetch and the checkout.
Git merge command
How do merge changes?
git merge command, merge change in the local repository with the working area. The command syntax is, git merge
How to merge two branches in git?
git merge command can also be used to merge one branch to another. Suppose you want to merge all commits in branch A to branch B. What you have to do is check out to branch B (git checkout command is used to change the current branch). Then run the git merge command.
-
Checkout to branch B:
git checkout B
-
Merge branch A to branch B:
git merge A
How to merge a commit from one branch to another?
Suppose you want to merge a specific commit in branch A to branch B. First checkout to branch B. Then run the git cherry-pick command with the commit_id to merge that commit from branch A to branch B.
-
Checkout to branch B:
git checkout B
-
Merge the commit in branch A to branch B:
git cherry-pick <commit_id>
How to discard a merge in git?
To discard a merge, first, get the commit hash before merging and then run the git reset command along with the commit hash.
-
Get commit hash before merging:
git log
-
Discard merge:
git reset --hard <commit_before_merge>
Git checkout command
The Git checkout command helps to switch among branches or discard given file(s).
-
Switch branch:
git checkout <branch_name>
-
Discard changes that you made to a specific file in the “working area”. In another way, this command discards changes before “add”:
git checkout <file_name>
-
Discard all changes that you made to more than one file in the “working area”:
git checkout .
-
Check out a new branch from the remote repository:
git checkout -b <remote_branch_name>
Git reset command
One usage of the reset command is to move changes from the Staging/indexing area to the working area.
-
Move specified file(s) from the Staging/indexing area to the working area:
git reset HEAD <file_name>
-
Move all files from the Staging/indexing area to the working area:
git reset HEAD
-
Move changes of a specified commit from the local repository to the working area:
git reset
-
Remove the last commit from the history and move changes in that commit from the local repository to the staging/indexing area:
git reset --soft <commit_hash>
-
Remove the last commit in the history and remove all the changes of that commit from the working area or from the indexing/stage area:
git reset --hard <commit_hash>
Git revert command
The git revert command is used to undo any commit by pushing a fresh commit into the remote repository. It doesn’t change the commit history but adds a fresh commit in the local repository.
- Remove changes from a given commit:
git revert <commit_id>
Git diff command
The Git diff command can be used to show current changes in your branch.
-
Shows current changes:
git diff
-
Show differences between a specific branch and the current head:
git diff <hash> HEAD
Tools available for git
Here you will see a few tools available for git. Those give you a user interface to work with Git. Git UI is very useful and saves your time when there are conflicts in your code and want to resolve them. Branch visualization is another advantage of those git tools.
- diffmerge
- Source tree
- GitLens extension for VS code
How to pull a git repo with large files?
Sometimes you may want to pull large files from remote repositories. When you try to pull repositories with large files, the pull process is getting failed. In that kind of situation, try to disable http.sslverify and try again with lfs option. lfs stands for large file storage.
git clone <remote_url>
cd <project_directory>
git config http.sslverify false (optional)
git lfs pull
TIPS
Quick steps to push changes into the remote repository
-
Shows all the changes that you have made:
git status
-
Move all the changes from the working area to the staging area:
git add .
-
Move all the changes from the staging area to the local repository area and update the history:
git commit -m "<message>"
-
Move changes from the local repository to the remote repository:
git push origin <branch_name>
Conclusion
In this guide, we discussed what is git and the areas in a git project on the local machine. Then we talked about basic git commands and tools that you can use to make life easy when working with Git.
FAQ
Q: Is Origin main local or remote?
A:Origin is pointing to remote git.
Q: What does git remote show origin do?
A:This is the result once you run the git remote show origin command.
remote origin
Fetch URL: https://SmadusankaB:[email protected]/SmadusankaB/react-refresh-page.git
Push URL: https://SmadusankaB:[email protected]/SmadusankaB/react-refresh-page.git
HEAD branch: main
Remote branch:
main tracked
Local branch configured for 'git pull':
main merges with remote main
Local ref configured for 'git push':
main pushes to main (up to date)
Q: What is Origin and Main in git push?
A:The origin is the short form of the URL of the remote server. Main is the first branch name of your repository. But you can give any for the first branch when you create the repository. Sometimes it is called master.
Q: What are the three main local areas in git?
A: Basically, there are three main areas called the working area, staging area/indexing area, and local repository. Apart from that, there is one more area called the stashed area.
Q: Should I use Git CLI or GUI?
A:If you are a programmer/Developer you must use git CLI because all environments don’t have git GUI installed. But git CLI is available everywhere. If it is not there you can install it very easily unlike installing GUI.
Comments
There are no comments yet.